Python has officially surpassed JavaScript as the most popular programming language, according to GitHub. Many attribute this rise to the powerful new upgrade—Python 3.13, featuring its JIT compiler. This leap in performance raises the question: Could this new feature establish Python as the most relevant programming language of 2025? Let’s explore!
What’s New In Python 3.13
Python 3.13 introduces a variety of updates that enhance performance, usability, and developer experience. Among the most significant changes is the experimental support for free-threaded execution, which allows Python to operate without the Global Interpreter Lock (GIL) on specific builds. This enables multi-threaded programs to fully utilize multiple CPU cores, although it remains experimental and may exhibit unexpected behavior. Developers can toggle this mode with specific binaries or environment variables.
On the other hand, the interactive interpreter has received substantial upgrades. It now supports multi-line editing, colorized prompts, and enhanced history navigation, making it easier to work with complex code. Features like "paste mode" streamline large code block input, while commands such as clear
and direct typing of help
or cgi, crypt,
and mailcap
simplify usability. These changes make Python's REPL (Read-Eval-Print Loop) more user-friendly.
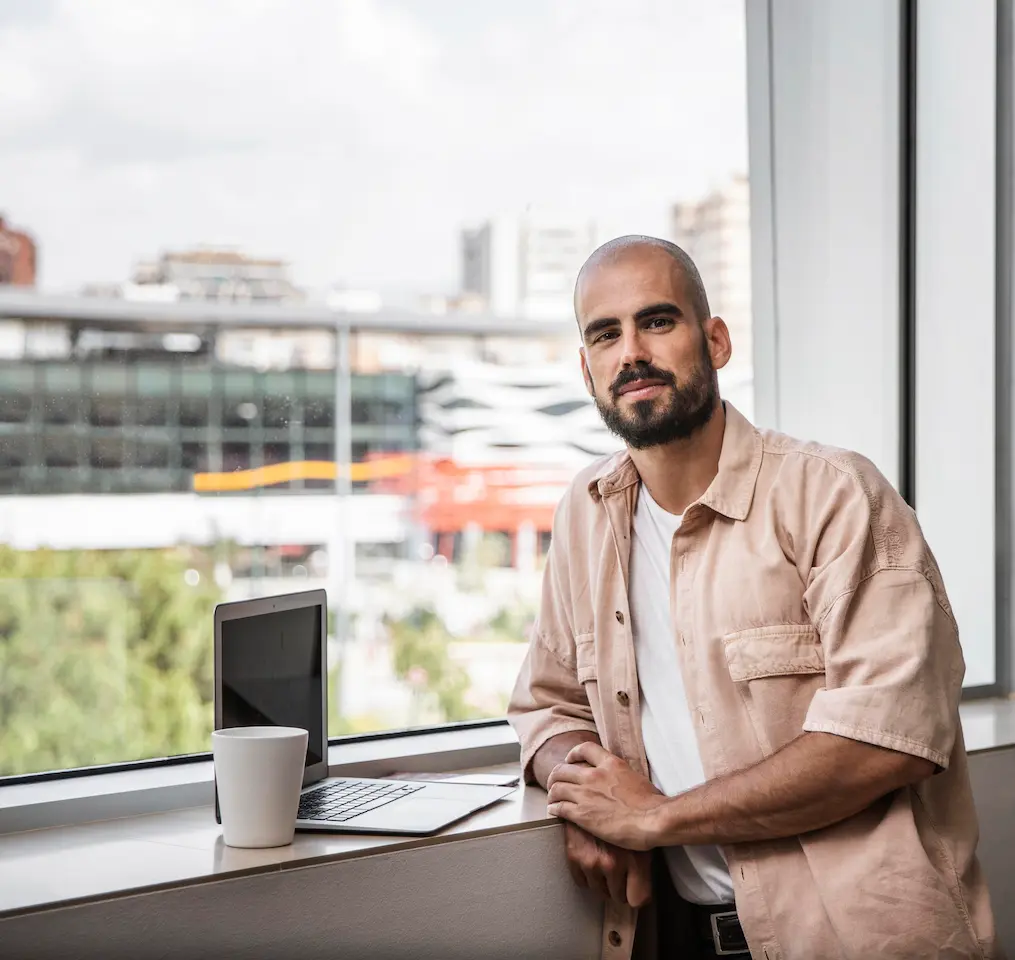
Additionally, an experimental Just-In-Time (JIT) compiler has been introduced, improving execution speed by compiling Python code into machine code during runtime. Though still in development and disabled by default, it represents a significant step forward for Python's performance capabilities, especially for compute-heavy tasks.
The update also includes enhanced error messages, which are now providing clearer and more actionable insights. For example, when a script name conflicts with a standard library module, Python suggests renaming it to avoid issues. Additionally, errors caused by incorrect keyword arguments now attempt to suggest the correct ones, improving debugging efficiency.
Other news includes changes in several standard libraries, such as the removal of outdated modules like cgi
, crypt
, and mailcap
as part of PEP 594. These "dead batteries" have been deprecated
to streamline the language. In addition, locals()
now returns an independent snapshot of variables, ensuring consistency in debugging scenarios.
Further enhancements include support for read-only fields in TypedDict, the introduction of a deprecated
decorator to mark outdated code, and memory optimizations for documentation strings. Lastly, Python 3.13 officially supports iOS and Android as Tier 3 platforms, expanding its cross-platform capabilities.
Free Threading and JIT in Python 3.13: What’s the Fuss?
Python 3.13 introduces two experimental features—free-threaded execution and Just-In-Time (JIT) compilation—both aimed at improving Python’s performance and scalability, particularly for multi-core systems and computationally intensive tasks.
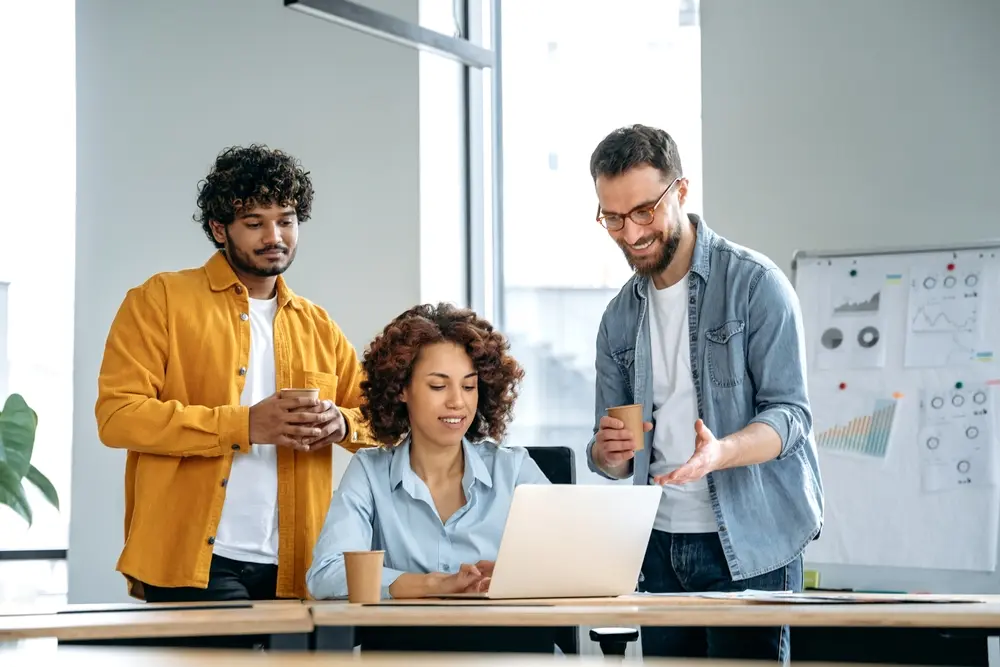
Free-Threaded Execution
Python 3.13 enables free-threaded execution by allowing the Global Interpreter Lock (GIL) to be disabled on specific builds. Traditionally, the GIL limited Python's ability to execute multiple threads in parallel, as it only permitted one thread to execute Python bytecode at a time. With this new capability, developers can fully utilize multi-core processors for true parallelism in multi-threaded applications. This change significantly boosts performance for workloads such as neural networks and numerical computations that benefit from parallelism. However, since this feature is experimental, it might introduce bugs or performance regressions when running on single-core systems. Developers can toggle the GIL using the -X gil=1
flag or environment variables like PYTHON_GIL.
Just-In-Time (JIT) Compilation
Python 3.13 also introduces an experimental JIT compiler designed to enhance runtime performance by translating Python bytecode into machine code dynamically. The JIT employs a "copy-and-patch" approach based on LLVM, a high-performance compiler infrastructure. While disabled by default, enabling the JIT during the build process can lead to faster program execution for certain workloads.
However, developers should note that the JIT is still in the early stages, and its benefits may not be universally evident. Current JIT implementations focus on maintaining backward compatibility and aim to improve both speed and memory usage in future iterations.
Both features represent major milestones for Python, aligning it with modern programming languages like Rust, Go, and Java, which already offer robust support for parallelism and just-in-time compilation. While these features remain experimental, they provide developers with new opportunities to explore enhanced performance in Python.
What is a JIT?
A Just-In-Time (JIT) compiler is a tool that improves program performance by converting code into machine language at runtime, rather than compiling it ahead of time (AOT). This dynamic compilation allows the program to optimize execution based on actual usage patterns, leading to faster execution speeds for many workloads.
In Python, JIT compilation translates Python bytecode into efficient machine code during program execution, potentially eliminating some of the overhead associated with Python's interpreted nature. This feature is particularly beneficial for tasks involving intensive computation or tight loops, where the speed-up from JIT-compiled code can be significant.
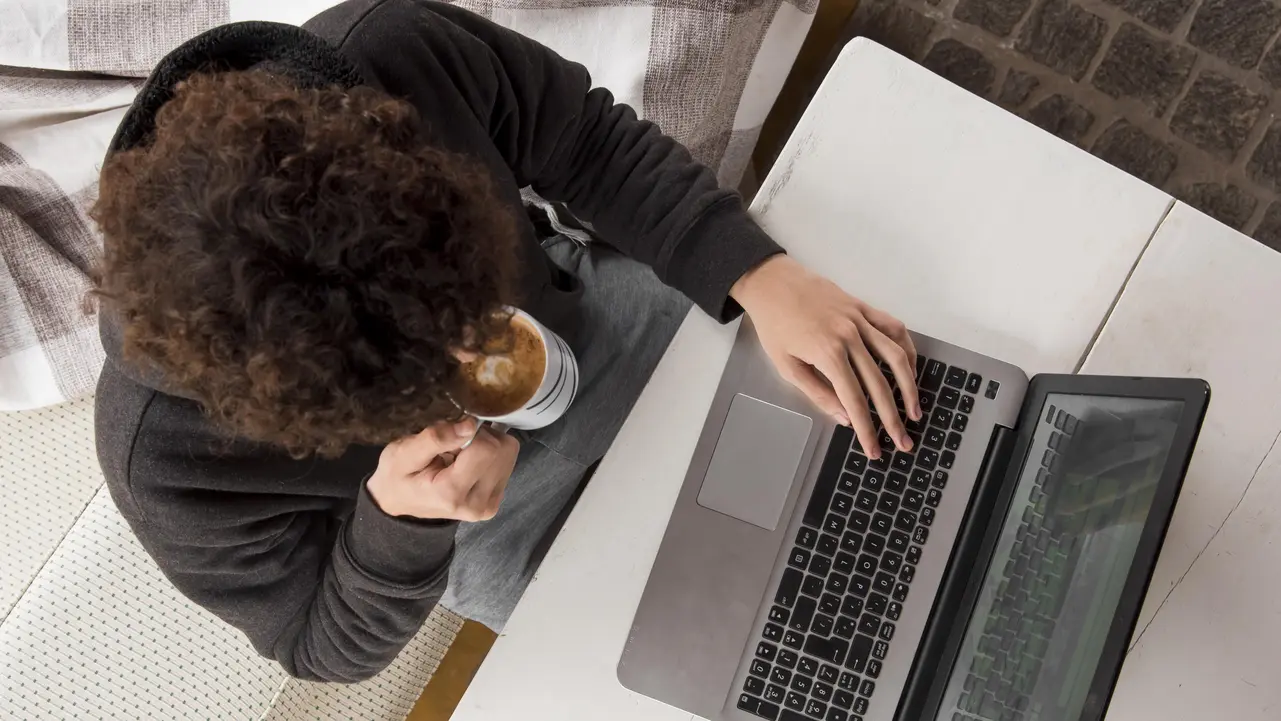
What is a copy-and-patch JIT?
A copy-and-patch JIT (Just-In-Time compiler) is a compilation strategy designed to optimize high-level language execution by dynamically converting bytecode into efficient machine code.
The "copy-and-patch" approach works by reusing precompiled code snippets from a library of binary implementations and then "patching" them to fit specific execution contexts at runtime. This technique is particularly effective for high-level languages like Python, where maintaining flexibility and compatibility with dynamic typing is critical.
Copy-and-patch JITs achieve faster compilation times while preserving performance gains through assembling machine code through lightweight modifications rather than building it from scratch. In Python 3.13, this method is part of the experimental JIT implementation, offering a balance of speed and adaptability, albeit with some additional memory and build-time requirements.
Why a copy-and-patch JIT?
A copy-and-patch JIT (Just-In-Time compiler) is well-suited for Python due to its focus on balancing performance, simplicity, and compatibility with Python's dynamic nature. This approach relies on precompiled binary templates for common operations and adjusts or "patches" them during runtime to fit the specific program context. This offers several advantages:
Performance Efficiency: By starting with precompiled templates, the copy-and-patch JIT avoids the time-intensive process of generating machine code entirely from scratch. This results in faster compilation times while still providing significant runtime performance improvements over traditional interpretation.
Adaptability to Python's Dynamics: Python's highly dynamic nature, including features like runtime type changes and flexible data structures, requires a JIT that can adapt quickly without losing efficiency. Copy-and-patch achieves this by applying runtime-specific modifications to general-purpose code snippets, ensuring compatibility with Python's diverse use cases.
Simplicity and Maintainability: Compared to more complex JIT designs, this approach is easier to implement and maintain. It allows for a more incremental development process while ensuring that the JIT integrates seamlessly into Python's existing ecosystem.
Backward Compatibility: By using precompiled templates and adapting them, this JIT design minimizes disruptions to existing Python code and ensures backward compatibility, a core requirement for Python's extensive user base.
This strategy aligns with Python’s goals of optimizing performance while preserving its ease of use and flexibility. As part of Python 3.13's experimental JIT feature, the copy-and-patch method is a step toward improving execution speed, especially for compute-heavy applications, while maintaining Python's hallmark simplicity.
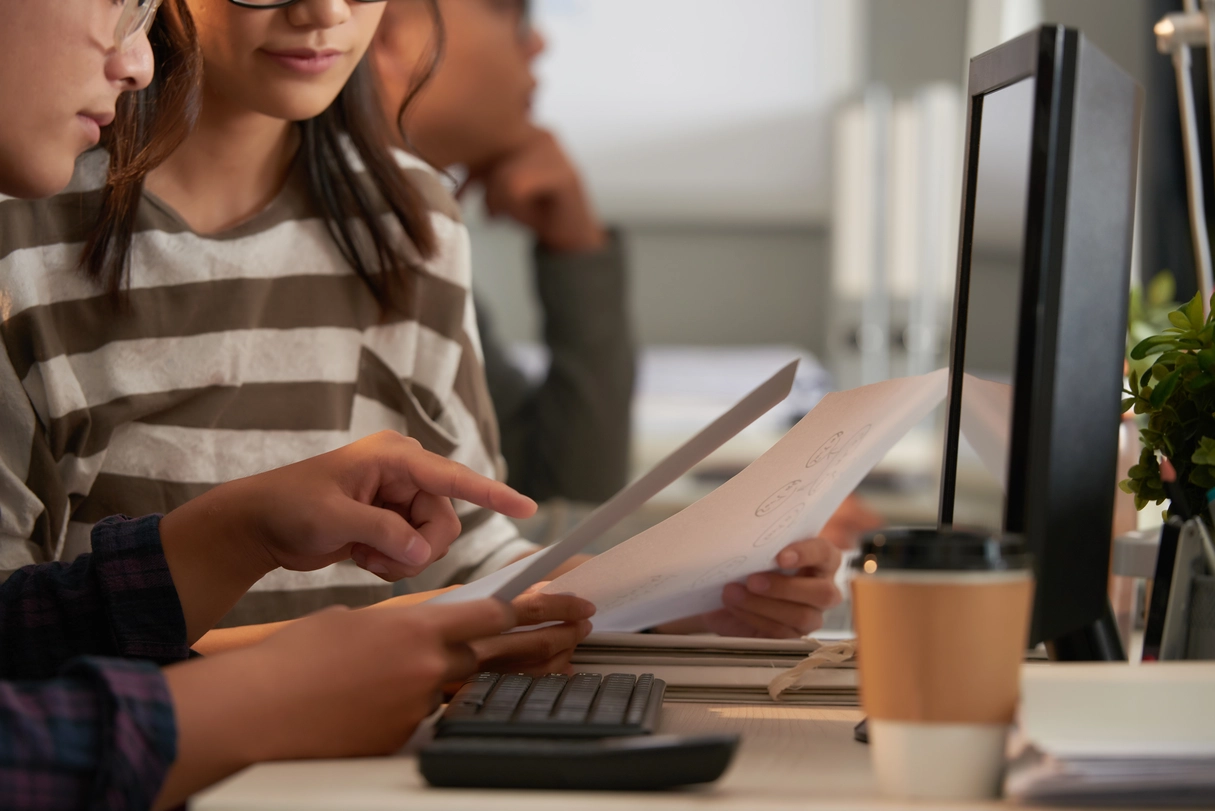
So how does this JIT work?
The copy-and-patch JIT (Just-In-Time compiler) in Python 3.13 improves runtime performance by dynamically converting Python bytecode into machine code while balancing speed and flexibility. It operates by using a library of precompiled machine code templates for common operations, such as arithmetic or function calls. These templates serve as reusable building blocks, allowing the JIT to avoid generating machine code from scratch, which would otherwise be time-consuming.
When executing Python code, the JIT selects a suitable template and modifies, or "patches," it to fit the specific runtime context. For example, the JIT might adapt the code based on the types of variables involved (e.g., integers or floats) or optimize frequently executed paths for better efficiency. This combination of copying existing optimized code and customizing it dynamically makes the JIT faster and more memory-efficient than traditional approaches.
This method is particularly effective for Python because it aligns with the language's dynamic and flexible nature. It allows for rapid execution of Python programs while maintaining compatibility with existing code. Although experimental in Python 3.13, this approach sets the stage for significant performance enhancements in future versions.
Is it faster?
The copy-and-patch JIT in Python 3.13 has the potential to make code execution faster, particularly for compute-intensive tasks and frequently executed operations. The JIT reduces the overhead of Python’s interpreted execution model by translating Python bytecode into optimized machine code at runtime. Instead of interpreting bytecode repeatedly, it generates machine code once and runs it directly on the CPU, which can significantly boost performance for tight loops, mathematical operations, and code with predictable patterns.
However, the performance gains depend on the workload and the specific code being executed. Since the JIT in Python 3.13 is still experimental and turned off by default, its implementation is not yet as advanced as those in other languages like Java or JavaScript. Early benchmarks indicate modest improvements in runtime for some use cases but show that performance might not yet match Python’s specialized optimizations in areas like numerical libraries (e.g., NumPy).
In its current state, the JIT complements Python’s existing performance strategies and serves as a foundation for more significant enhancements in future releases. Its real-world impact will depend on continued development and optimization.
Other Pretty Cool Features
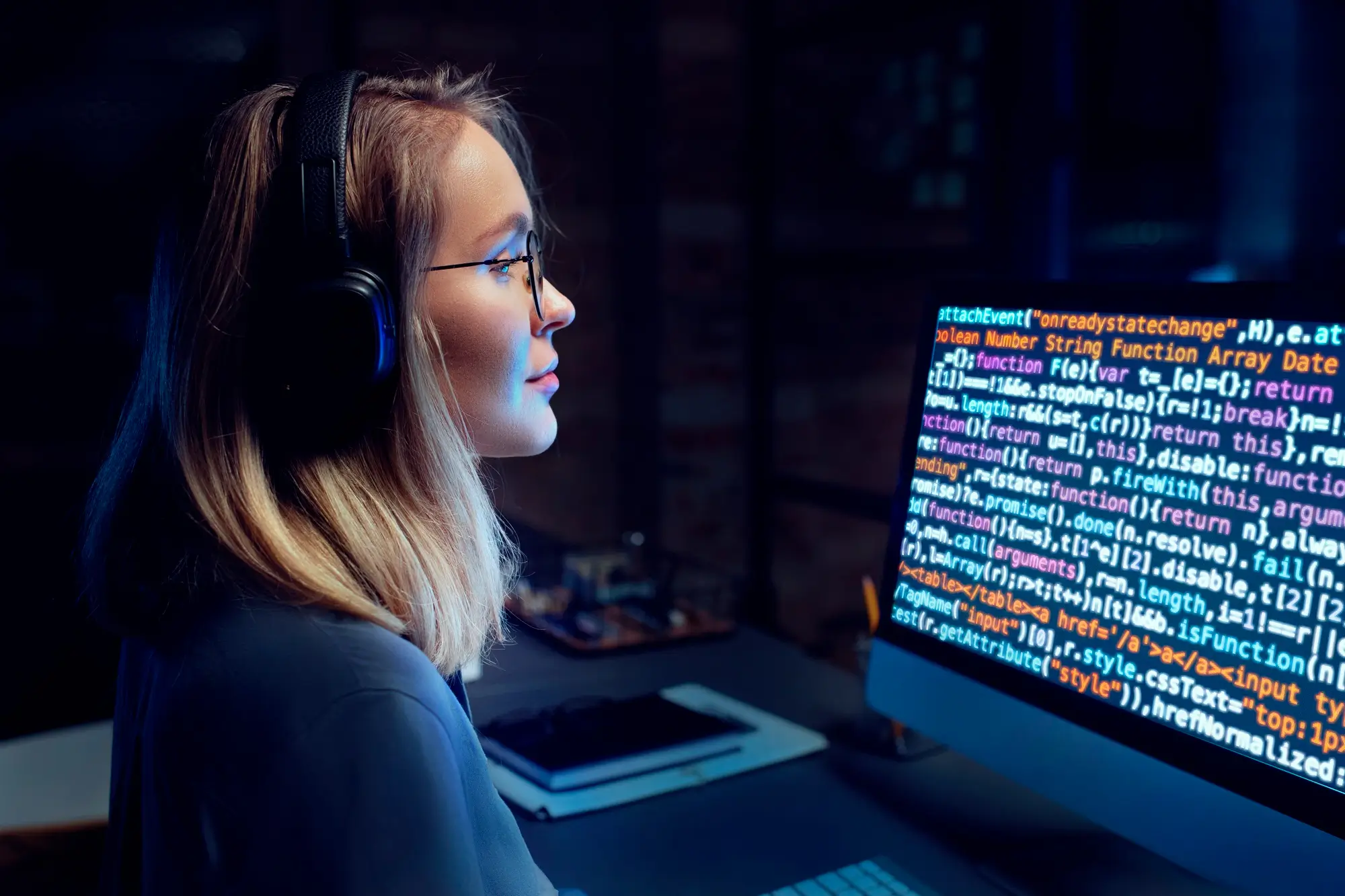
New Modules
In Python 3.13, the dbm.sqlite3
module introduces an SQLite-based backend to the dbm
interface as a new module, combining the simplicity of key-value storage with the robustness of SQLite. This new backend allows developers to use the familiar dbm
interface while leveraging SQLite's lightweight, reliable, and ACID-compliant database engine.
As part of Python’s standard library, dbm.sqlite3
is cross-platform and requires no additional setup, ensuring seamless use across different environments.
Key features of dbm.sqlite3
include efficient storage and retrieval of key-value pairs, making it ideal for simple databases, caching mechanisms, or configuration systems. By using SQLite as the underlying storage, the module benefits from its ability to handle larger datasets, indexes, and advanced storage requirements compared to traditional dbm
backends.
Additionally, dbm.sqlite3
avoids many of the size limitations and format restrictions of older dbm
backends while maintaining compatibility with the existing dbm
API, allowing developers to easily adopt this new backend in their applications.
Improved Modules
While Python 3.13 does not introduce many new modules, it delivers significant enhancements to existing ones, improving functionality, usability, and performance.
One significant update is to the asyncio
module, which now introduces Task Groups. Task Groups provide a structured way to manage multiple asynchronous tasks concurrently.
This addition improves error propagation, as exceptions raised in child tasks are automatically collected and handled, reducing the risk of silent failures. By grouping related tasks, developers can write cleaner and more reliable asynchronous code, simplifying the management of complex workflows.
The typing
module also receives notable enhancements, including expanded support for TypeGuard. This feature allows developers to define custom logic for narrowing types during runtime, enabling more precise and flexible type checking.
These improvements benefit large projects by enhancing static analysis, improving code readability, and supporting advanced use cases for type annotations. Additionally, the update includes better tooling integration, which strengthens Python's appeal for developers working in type-heavy domains like enterprise applications and machine learning.
Other modules, such as subprocess
and logging
, have seen smaller updates that improve developer ergonomics. For example, subprocess
gains more robust handling of asynchronous subprocess management while logging
offering refined options for customization and formatting. These changes, though incremental, make working with these modules more efficient and developer-friendly.
Overall, the improvements in Python 3.13's existing modules reflect the language's commitment to maintaining its relevance and usability while addressing the needs of its growing community. These updates make Python more powerful and flexible, especially for developers building asynchronous or type-driven applications.
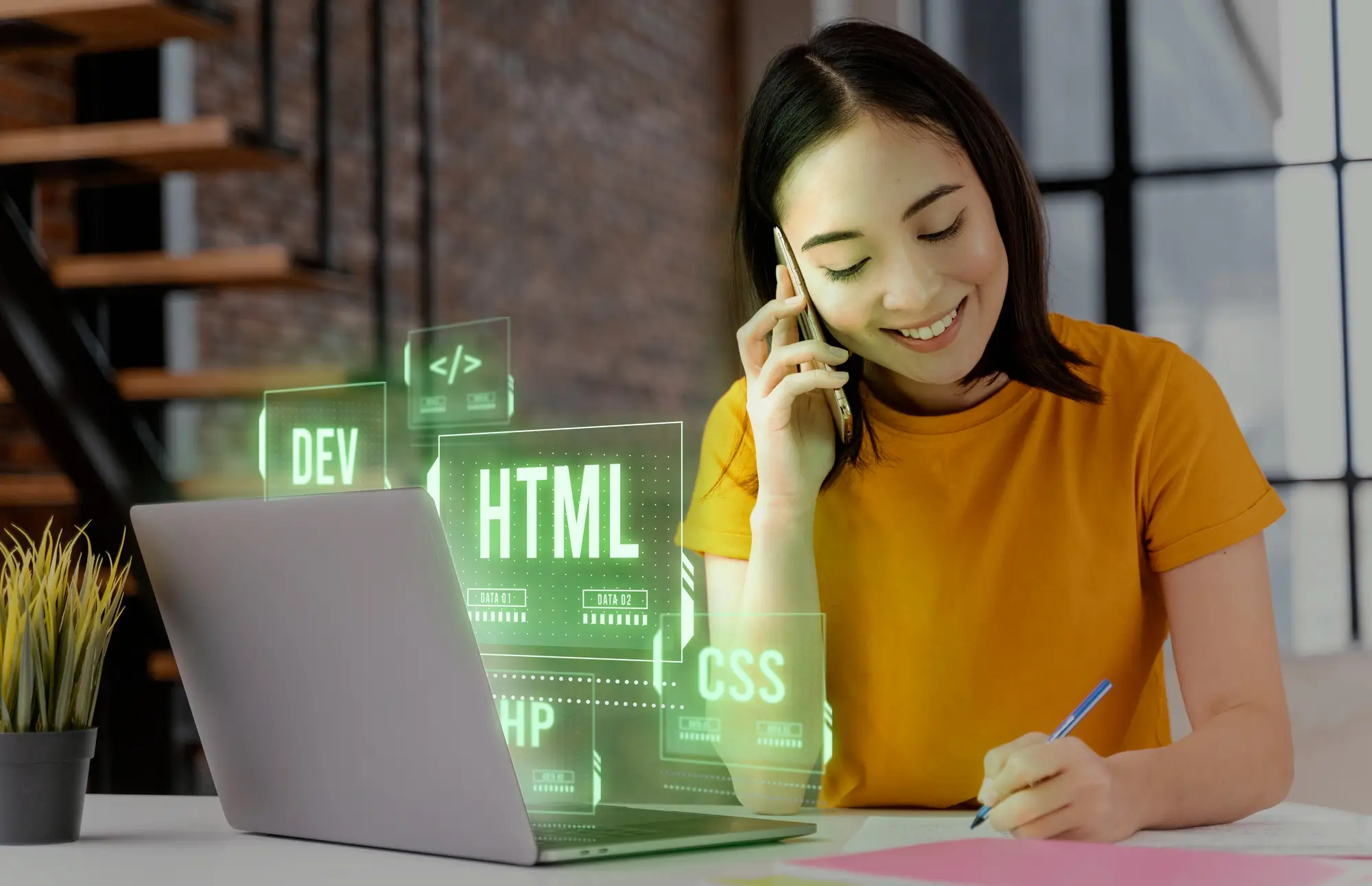
Regression Test Changes
Python 3.13 also introduces updates to its regression testing framework and approach, emphasizing improved reliability, expanded coverage, and enhanced debugging tools. Regression tests, which ensure that new changes or features do not break existing functionality, are critical to maintaining Python's stability across its vast codebase.
One significant change is the enhancement of test
modules within the standard library, particularly in terms of usability and reporting. Python 3.13 improves test output clarity, making it easier to identify failures and pinpoint their root causes.
This is especially helpful when working with large test suites, as clearer diagnostics can save developers significant time during debugging.
Additionally, Python's test framework now includes better support for testing platform-specific features and configurations, which ensures that Python remains stable across a wider range of environments, including less common platforms.
For instance, improvements in automated tests for Tier 3 platforms like iOS and Android allow for more thorough validation, which is crucial as Python expands its support to these platforms.
Finally, Python 3.13 introduces refined tools for mocking, stubbing, and patching during tests. This makes it easier for developers to isolate specific components, test edge cases, and simulate various runtime conditions without depending on external resources. These changes further bolster Python’s testing capabilities, enabling more robust and granular regression tests.
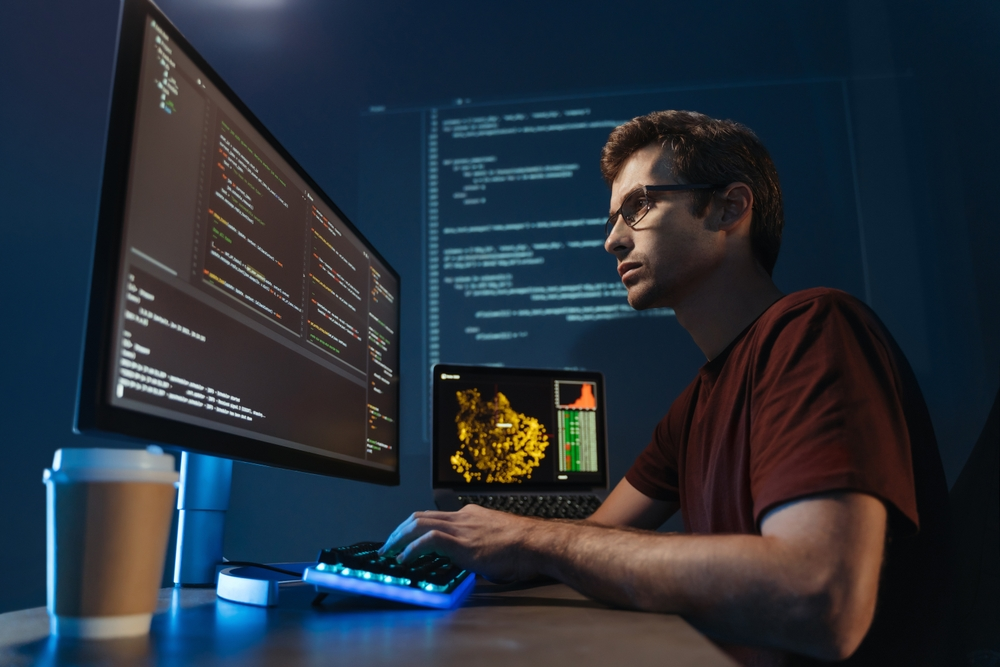
So, Should You Upgrade to Python 3.13?
Whether you should upgrade to Python 3.13 depends on your specific use case and environment, but it’s worth considering if you value the new features and improvements it brings. Python 3.13 includes experimental features like free-threaded execution (disabling the GIL) and Just-In-Time (JIT) compilation, which promise significant performance gains for multi-threaded and compute-intensive applications.
If you are a JIT compiler expert, you will find these enhancements promising, moving Python closer to bridging the performance gap with lower-level languages. Though experimental, the JIT and free-threaded execution in Python 3.13 can significantly boost CPU-intensive and multi-threaded tasks. For organizations invested in Java Development Services, integrating these new Python capabilities could create a more flexible, high-performing environment—just be sure to thoroughly test them before production.
However, these features are still experimental and may not yet provide consistent performance improvements across all workloads. For developers focused on asynchronous programming or type hinting, Python 3.13 offers substantial enhancements. Improvements to the asyncio
module, such as Task Groups, make working with concurrent tasks more efficient and structured. Similarly, better support for TypeGuard
in the typing
module enhances type-checking capabilities, particularly for large, complex codebases. If these features align with your projects, upgrading could boost productivity and code quality.
On the other hand, some deprecated modules have been removed (e.g., cgi, crypt, mailcap
), which might impact older codebases. If your project relies on these modules or third-party packages that have not yet been updated for compatibility with Python 3.13, you might want to delay the upgrade.
For most users, especially those running modern, actively maintained applications, Python 3.13 is a step forward in performance and usability. However, given the experimental nature of its standout features, you should carefully test your codebase in a Python 3.13 environment before committing to an upgrade. If your workflow does not benefit directly from the new features, you can afford to wait for more stability and adoption in future releases.